Serverless with Lambda: Automate Operations by Auto-Stopping EC2 Instances
- Janice Sanders
- Jan 25, 2024
- 3 min read
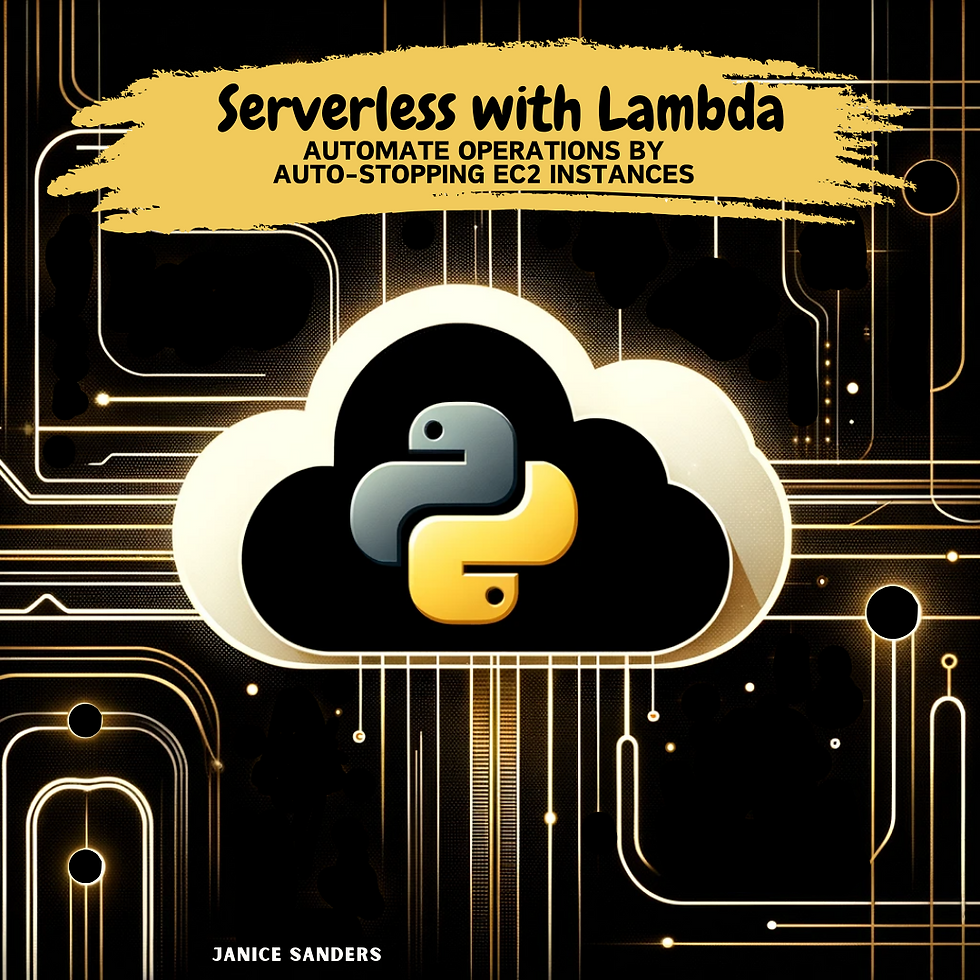
Managing cloud resources efficiently is key to controlling operational costs. Using serverless resources, such as Lambdas, are a great way to automate operations and optimize costs.
As a Cloud Engineer on the DevOps team, you must come up with efficient ways to utilize compute resources in the AWS cloud environment. The team needs to ensure that any running EC2 instances in the development lab are stopped by the end of day. To address this, we developed a solution to automatically stop all running EC2 instances after work hours at 7 pm.
I’ve outlined how we achieved this automated solution utilizing an AWS Lambda and python.
1️⃣Create the Lambda Function
2️⃣Add the Python Code to the Lambda
3️⃣Add a Trigger to the Lambda Function
4️⃣Configure the IAM Role with Appropriate Permissions
5️⃣Test the Lambda Function
6️⃣Validate the Lambda Function Actions
7️⃣Commit the Python Code to GitHub
🏴To get started, here are some helpful prerequisites:
🔸AWS Account: An active AWS account with knowledge of IAM permissions to manage compute resources, including EC2 instances and AWS Lambda.
🔸AWS Test Environment: Access to a non-production AWS environment for safely testing the Lambda function.
🔸Python and Boto3 Knowledge: Basic understanding of Python scripting and familiarity with the Boto3 library for interacting with AWS services.
🔸IDE Tool: Access to a text editor or IDE for writing Python code, such as Cloud9 or Visual Studio Code.
🔸GitHub Account: Knowledge of GitHub for version control and code management.
Start by creating the lambda function, which is the core of the automation process.
1️⃣Create the Lambda Function:
2️⃣Add the python code to the ‘Code’ section of the lambda function:
The code will import AWS SDK boto3 for Python.
import boto3 # Import AWS SDK for Python
def lambda_handler(event, context):
# Entry point for the Lambda function
stop_ec2_instances() # Call the function to stop EC2 instances
def stop_ec2_instances():
ec2 = boto3.client('ec2') # Initialize EC2 client
# Retrieve all instances in 'running' state
instances = ec2.describe_instances(
Filters=[{'Name': 'instance-state-name', 'Values': ['running']}]
)
# Extracting instance IDs from the response
running_instances = [instance['InstanceId'] for reservation in instances['Reservations'] for instance in reservation['Instances']]
if running_instances:
# If there are running instances, print their IDs and issue a stop command
print(f"Stopping instances: {running_instances}")
ec2.stop_instances(InstanceIds=running_instances)
else:
# If no running instances are found, log this information
print("No running instances found.")
# Note: The function logs will be available in AWS CloudWatch under the /aws/lambda/<function-name> log group.
The python code is added to the lambda_function.py file.
3️⃣Add a Trigger to the Lambda Function:
Add a trigger that runs on a set schedule each day. The trigger is an EventBridge (CloudWatch Events) rule that stops all running ec2 instances in the environment at 7 pm.
4️⃣Configure the IAM Role with Appropriate Permissions:
The IAM role associated with the lambda function should have permissions to execute the Lambda, as well as permissions to describe and stop the ec2 instances.
5️⃣Test the Lambda Function:
As a sanity check to test the lambda function, I have confirmed that there is only one running instance in the environment.
After triggering the lambda function, it shows that the instance is stopping. The code has been written to reference the Instance IDs for all stopped instances.
6️⃣Validate the Lambda Function Actions:
To validate, I rechecked the instance state of the referenced ec2 instance. There are now no running instances in the environment.
The instance has indeed been stopped by the lambda function. 👏
7️⃣Commit the Python Code to GitHub:
After testing that the lambda is functional, I pushed the code to GitHub via Visual Studio Code for easy accessibility and collaboration.
The script is accessible here on GitHub: Teach2Geek GitHub
This automated solution ensures that EC2 instances are not left running unnecessarily outside of working hours. It demonstrates how automation can be leveraged for cost efficiency in DevOps environments.
Feel free to use and modify, as needed, to accommodate your environment.
To receive my latest projects, playbooks, and posts, follow my Medium page, and Subscribe to get email notifications when I post new stories.
For a more personal connection, connect with me on LinkedIn to network and grow together. 🔗
Comments